Craft Scalable Apps with
Angular
Sharpen Skills, Ace Interviews
Backend Development Interview Questions For Angular
Angular is a modern web application framework developed by Google. It is a complete rewrite of AngularJS, designed to build dynamic single-page applications (SPAs). Angular uses TypeScript, a superset of JavaScript, and offers better performance, modularity, and a more structured approach compared to AngularJS. Angular also has a component-based architecture, while AngularJS relies on a scope-based model.
Components are the building blocks of an Angular application. They consist of a TypeScript class that manages data and logic, an HTML template that defines the view, and optionally, CSS for styling. Each component is a self-contained unit, and an Angular application is typically composed of many components working together.
Example:
TypeScript:
import { Component } from '@angular/core';
@Component({
selector: 'app-hello',
template: '<h1>Hello, {{ name }}!</h1>',
styleUrls: ['./hello.component.css']
})
export class HelloComponent {
name: string = 'Angular';
}
Angular CLI (Command Line Interface) is a powerful tool that simplifies Angular development by providing commands to create, build, test, and deploy Angular applications. It automates many common tasks like setting up a project, adding components, and managing dependencies, which helps developers follow best practices and reduces the complexity of manual configuration.
Example:
bash:
ng new my-angular-app # Create a new Angular project
ng serve # Run the development server
ng generate component my-component # Generate a new component
Angular modules (NgModules) are containers that group related components, directives, pipes, and services. They help organize an application into cohesive blocks of functionality. The root module, AppModule, is required for bootstrapping the application. Additional feature modules can be created to encapsulate specific functionality.
Example:
TypeScript:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { HelloComponent } from './hello.component';
@NgModule({
declarations: [AppComponent, HelloComponent],
imports: [BrowserModule],
bootstrap: [AppComponent]
})
export class AppModule {}
Angular provides several types of data binding to synchronize data between the model and the view:
- Interpolation: {{ expression }} - One-way binding from the component to the view.
- Property Binding: [property]="expression" - One-way binding from the component to the element's property.
- Event Binding: (event)="handler" - One-way binding from the element's event to the component's method.
- Two-way Binding: [(ngModel)]="property" - Two-way binding that combines property and event binding to keep the view and model in sync.
Example:
html:
<input [(ngModel)]="name"> <!-- Two-way binding -->
<p>Hello, {{ name }}!</p> <!-- Interpolation -->
A service in Angular is a class that provides reusable logic and data across different components. Services are typically used for tasks like data fetching, logging, and authentication. Services are injected into components or other services using Angular's dependency injection system.
Example:
TypeScript:
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root',
})
export class DataService {
getData() {
return ['Data1', 'Data2', 'Data3'];
}
}
Dependency injection (DI) in Angular is a design pattern where dependencies (services or objects) are provided to a class instead of being created by the class itself. Angular’s DI system automatically provides the required dependencies when a component or service is instantiated, promoting modularity and ease of testing.
Example:
TypeScript:
import { Component } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-data',
template: '<ul><li *ngFor="let item of data">{{ item }}</li></ul>',
})
export class DataComponent {
data: string[];
constructor(private dataService: DataService) {
this.data = this.dataService.getData();
}
}
Directives in Angular are special classes that modify the behavior of elements in the DOM. There are three types of directives:
- Component Directives: They are directives with a template (components are a type of directive).
- Structural Directives: They change the structure of the DOM, e.g., *ngIf, *ngFor.
- Attribute Directives: They change the appearance or behavior of an element, e.g., ngClass, ngStyle.
Example of a Structural Directive:
html:
<div *ngIf="isVisible">This is visible</div>
Angular’s routing module allows you to define and manage navigation between different views or pages in your application. Routes are configured in the AppRoutingModule, mapping URL paths to components. The RouterModule provides directives and services to handle navigation and route parameters.
Example:
TypeScript:
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { HomeComponent } from './home/home.component';
import { AboutComponent } from './about/about.component';
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent },
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule],
})
export class AppRoutingModule {}
Lifecycle hooks are methods in an Angular component or directive that are called at specific points in the lifecycle of the component or directive. These hooks allow you to perform custom logic during different phases of the component's lifecycle.
Some common lifecycle hooks:
- ngOnInit: Called once the component is initialized.
- ngOnChanges: Called when input properties change.
- ngOnDestroy: Called just before the component is destroyed.
Example:
TypeScript:
import { Component, OnInit, OnDestroy } from '@angular/core';
@Component({
selector: 'app-lifecycle',
template: '<p>Lifecycle Demo</p>',
})
export class LifecycleComponent implements OnInit, OnDestroy {
ngOnInit() {
console.log('Component Initialized');
}
ngOnDestroy() {
console.log('Component Destroyed');
}
}
Angular’s change detection mechanism automatically updates the view whenever the application state changes. Angular uses a unidirectional data flow and checks for changes by comparing the current and previous state of the component's data. The change detection process can be controlled and optimized using strategies like Default and OnPush.
Example:
TypeScript:
import { Component, ChangeDetectionStrategy } from '@angular/core';
@Component({
selector: 'app-onpush',
template: '<p>{{ data }}</p>',
changeDetection: ChangeDetectionStrategy.OnPush
})
export class OnPushComponent {
data: string = 'Initial Data';
}
Reactive Forms in Angular provide a more robust and scalable approach to handling form inputs compared to Template-driven forms. Reactive Forms are model-driven, where the form structure is defined in the component class using FormGroup, FormControl, and FormArray. This approach allows for better validation, testing, and dynamic form control.
Example:
TypeScript:
import { Component } from '@angular/core';
import { FormGroup, FormControl } from '@angular/forms';
@Component({
selector: 'app-reactive-form',
template: `
<form [formGroup]="form">
<input formControlName="name">
</form>
`,
})
export class ReactiveFormComponent {
form = new FormGroup({
name: new FormControl(''),
});
}
To optimize an Angular application, you can:
- Use Lazy Loading: Load feature modules only when needed.
- AOT Compilation: Use Ahead-of-Time (AOT) compilation to improve load time.
- OnPush Change Detection: Use OnPush strategy to reduce change detection cycles.
- Tree Shaking: Remove unused code with the help of build tools.
- Optimizing Bundle Size: Minify and compress files, and remove unnecessary dependencies.
Example of Lazy Loading:
TypeScript:
const routes: Routes = [
{
path: 'feature',
loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule)
}
];
Angular's HttpClient is a modern HTTP client that provides a more powerful and flexible API for making HTTP requests. It is an improvement over the old HttpModule, offering better type safety, improved error handling, and the ability to handle JSON responses by default. It also includes features like interceptors, which allow you to modify requests or responses globally.
Example:
TypeScript:
import { HttpClient } from '@angular/common/http';
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root',
})
export class DataService {
constructor(private http: HttpClient) {}
fetchData() {
return this.http.get('https://api.example.com/data');
}
}
RouterOutlet is a directive used in Angular's routing system to mark where the router should display the component for the current route. When the user navigates to a route, the RouterOutlet will load the corresponding component inside it.
Example:
html:
<router-outlet></router-outlet> <!-- Place where routed component will be displayed -->
Route guards in Angular are used to control access to routes based on certain conditions. There are several types of route guards, such as CanActivate, CanDeactivate, Resolve, CanLoad, and CanActivateChild. Implementing a route guard involves creating a service that implements one of these interfaces and returns a boolean or observable.
Example:
TypeScript:
import { Injectable } from '@angular/core';
import { CanActivate, Router } from '@angular/router';
@Injectable({
providedIn: 'root',
})
export class AuthGuard implements CanActivate {
constructor(private router: Router) {}
canActivate(): boolean {
const isAuthenticated = /* check authentication logic */;
if (!isAuthenticated) {
this.router.navigate(['/login']);
return false;
}
return true;
}
}
The async pipe in Angular is a pipe that subscribes to an observable or promise and returns the latest value it has emitted. When the observable emits a new value, the pipe updates the view automatically. The async pipe also handles unsubscribing when the component is destroyed.
Example:
html:
<div *ngIf="data$ | async as data">
<p>{{ data }}</p>
</div>
Form validation in Angular can be done using either Template-driven or Reactive Forms. Angular provides built-in validators like required, minLength, and pattern, and also allows you to create custom validators.
Example with Reactive Forms:
TypeScript:
import { FormGroup, FormControl, Validators } from '@angular/forms';
const form = new FormGroup({
name: new FormControl('', [Validators.required, Validators.minLength(3)]),
});
Example with Template-driven Forms:
HTML:
<form #form="ngForm">
<input name="name" ngModel required minlength="3">
</form>
Pipes in Angular are used to transform data before displaying it in the view. Angular comes with several built-in pipes like DatePipe, CurrencyPipe, and UpperCasePipe, but you can also create custom pipes.
Example of a custom pipe:
TypeScript:
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({ name: 'exclaim' })
export class ExclaimPipe implements PipeTransform {
transform(value: string): string {
return value + '!';
}
}
Usage in template:
HTML:
<p>{{ 'Hello' | exclaim }}</p> <!-- Outputs: Hello! -->
Internationalization (i18n) in Angular refers to the process of making your application adaptable to different languages and regions. Angular provides tools and techniques to handle translations, date formats, number formats, and more. The i18n attribute in templates is used to mark translatable text, and Angular's @angular/localize package is used for localization.
Example:
HTML:
<p i18n>Hello, world!</p>
- ng-template: A template element that defines a block of HTML that can be instantiated dynamically. It is not rendered directly but can be used with structural directives.
- ng-container: A logical container that doesn't render any DOM but can be used to group elements without introducing extra markup.
- ng-content: Used for content projection, allowing you to pass content from a parent component to a child component's template.
Example of ng-template:
HTML:
<ng-template #myTemplate>
<p>This is a template content</p>
</ng-template>
Error handling in Angular can be done globally using ErrorHandler, a service that allows you to handle all errors in one place, or locally within components or services. Additionally, HTTP errors can be managed using interceptors.
Example:
TypeScript:
import { ErrorHandler, Injectable } from '@angular/core';
@Injectable()
export class GlobalErrorHandler implements ErrorHandler {
handleError(error: any): void {
// Handle the error here
console.error('An error occurred:', error);
}
}
@keyframes example {
from {background-color: red;}
to {background-color: yellow;}
}
div {
animation: example 5s infinite;
}
Lazy loading in Angular allows you to load feature modules only when they are needed, reducing the initial load time. This is achieved by configuring the routes using the loadChildren property.
Example:
TypeScript:
const routes: Routes = [
{
path: 'feature',
loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule)
}
];
A resolver in Angular is a service that pre-fetches data before a route is activated. It ensures that the required data is available before the component is rendered. Resolvers are implemented using the Resolve interface.
Example:
TypeScript:
import { Resolve, ActivatedRouteSnapshot, RouterStateSnapshot } from '@angular/router';
import { Injectable } from '@angular/core';
import { Observable } from 'rxjs';
import { DataService } from './data.service';
@Injectable({
providedIn: 'root',
})
export class DataResolver implements Resolve<any> {
constructor(private dataService: DataService) {}
resolve(route: ActivatedRouteSnapshot, state: RouterStateSnapshot): Observable<any> {
return this.dataService.fetchData();
}
}
// Route configuration
const routes: Routes = [
{
path: 'data',
component: DataComponent,
resolve: { data: DataResolver },
},
];
Renderer2 is a service in Angular that allows you to manipulate DOM elements in a platform-agnostic way. It is preferred over directly interacting with the DOM to ensure compatibility across different platforms, like server-side rendering.
Example:
TypeScript:
import { Component, Renderer2, ElementRef } from '@angular/core';
@Component({
selector: 'app-renderer-demo',
template: '<div></div>',
})
export class RendererDemoComponent {
constructor(private renderer: Renderer2, private el: ElementRef) {
this.renderer.setStyle(this.el.nativeElement, 'color', 'blue');
}
}
ViewChild is a decorator in Angular that allows you to access a child component, directive, or DOM element from the parent component. It is used to interact with child elements and components after the view has been initialized.
Example:
TypeScript:
import { Component, ViewChild, ElementRef } from '@angular/core';
@Component({
selector: 'app-parent',
template: '<input #inputElement>',
})
export class ParentComponent {
@ViewChild('inputElement') input: ElementRef;
ngAfterViewInit() {
this.input.nativeElement.focus(); // Set focus on the input element
}
}
import { Component, Input, OnChanges, SimpleChanges } from '@angular/core';
@Component({
selector: 'app-child',
template: '<p>{{ data }}</p>',
})
export class ChildComponent implements OnChanges {
@Input() data: string; // Input property to receive data from the parent component
ngOnChanges(changes: SimpleChanges) {
if (changes['data']) {
console.log('Data changed:', changes['data'].currentValue);
}
}
}
ChangeDetectorRef is a service that allows you to manually control change detection in Angular. It is useful when you need to detect changes outside of Angular’s automatic change detection mechanism. You can use methods like markForCheck(), detectChanges(), and checkNoChanges() to manage change detection manually.
Example:
TypeScript:
import { Component, ChangeDetectorRef, OnInit } from '@angular/core';
@Component({
selector: 'app-manual-detection',
template: '<p>{{ data }}</p>',
})
export class ManualDetectionComponent implements OnInit {
data: string = 'Initial Data';
constructor(private cdRef: ChangeDetectorRef) {}
ngOnInit() {
setTimeout(() => {
this.data = 'Updated Data';
this.cdRef.detectChanges(); // Manually trigger change detection
}, 1000);
}
}
Angular Guards are used to control navigation within an application. CanDeactivate is a type of route guard that can prevent a user from navigating away from a component if certain conditions are met. It is typically used to warn users about unsaved changes or prevent navigation under specific conditions.
Example:
TypeScript:
import { Injectable } from '@angular/core';
import { CanDeactivate } from '@angular/router';
@Injectable({
providedIn: 'root',
})
export class CanDeactivateGuard implements CanDeactivate<CanComponentDeactivate> {
canDeactivate(component: CanComponentDeactivate): boolean {
return component.canDeactivate ? component.canDeactivate() : true;
}
}
export interface CanComponentDeactivate {
canDeactivate: () => boolean;
}
Usage in Component:
TypeScript:
import { Component } from '@angular/core';
@Component({
selector: 'app-edit',
template: '<p>Edit your data here</p>',
})
export class EditComponent implements CanComponentDeactivate {
canDeactivate(): boolean {
return confirm('Do you want to discard changes?');
}
}
Angular provides a robust animation module that allows you to create complex animations using the @angular/animations package. You can define animations using Angular's trigger, state, style, animate, and transition functions. Animations are typically used to enhance user experience by providing visual feedback.
Example:
TypeScript:
import { Component } from '@angular/core';
import { trigger, state, style, animate, transition } from '@angular/animations';
@Component({
selector: 'app-fade',
template: '<div [@fadeInOut]="state" (click)="toggleState()">Click me!</div>',
styles: [':host { display: block; margin: 20px; }'],
animations: [
trigger('fadeInOut', [
state('void', style({ opacity: 0 })),
state('*', style({ opacity: 1 })),
transition('void <=> *', animate(300)),
]),
],
})
export class FadeComponent {
state: string = 'visible';
toggleState() {
this.state = this.state === 'visible' ? 'hidden' : 'visible';
}
}
Example of a basic Flexbox layout:
.navbar {
display: flex;
justify-content: space-between;
}
@media (max-width: 600px) {
.navbar {
flex-direction: column;
}
}
Working Areas
IT Services Coustomized for your Industry
Website
Web application
Technology
Android
Designing
Solution
Company Benefits
We’re more than an agency
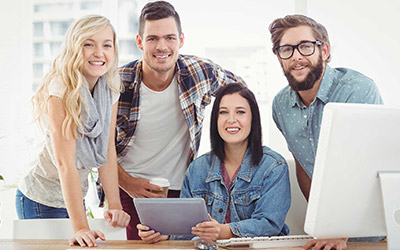
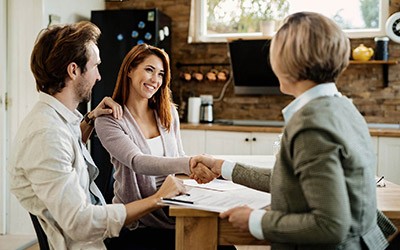
Best user interfaces
Quality web design
Is my technology allowed on tech?
How to soft launch your business?
What makes your business plans so special?
What industries do you specialize in?
What makes your business plans so special?
Completely iterate covalent strategic theme
Get in touch
We are here to help you & your business
Timings
9:00 am - 5:00 pm